Aligning 3d objects with Python for architectural modeling
The use of scripts to create routines and do repetitive tasks in 3d modeling is a common practice among more experienced artists, and for architectural modeling it's no different. In Blender 3D we use Python to create scripts that can help us in the modeling process. From time to time, I show a few scripts created by talented artists or programmers that help a lot in the modeling process. But, what if we wanted to create our own scripts? Today I will start a series of articles, showing how you can create a few simple scripts that will help in the modeling process for architecture.
You can use those scripts as a starting point to write your own tools and improve your workflow. The first script will deal with the alignment of objects. For instance, we can take several furniture models and set them aligned with another object, with a common tool available in 2d drawing tools such as Inkscape, Corel Draw or Photoshop.
To get started, we have to first setup our scene and add a Text Editor window in Blender 3D. For this tutorial I will be using Blender 3d 2.49b and Python 2.6.2. In the scene I have added several cubes, named with Blender 3d default names starting with Cube and finishing with Cube.005.
The first thing we have to do to create a script that deals with Blender 3d objects is import the modules of Blender to use in the Script. We do that with and “Import Blender” at the first line.
After that, we will now be able to access objects on the 3d view. For instance, if we use the Blender.Object.Get(‘name') command, and add the name of any object between the ”, the object properties will be available to use and mix with other pieces of code. For instance, we can create an object that will be used to represent the model pointed in the Get. I will create an object called myObject. The code will look like this:
- import Blender
- myObject = Blender.Object.Get(‘Cube')
With this code, I'm capturing the properties of the object named Cube and assigning it to the myObject. If we want to test if the code is right, just add a print command with the name of the object.
- import Blender
- myObject = Blender.Object.Get(‘Cube')
- print myObject
If we press ALT+P or use the Run Python script in the file menu of the Text Editor, we should see a message displayed at the auxiliary window.
The message shows [Object Cube], which shows that our script is working.
Now, we can transform the object any way we like. For instance, if we want to move the object to the point 30 in X, just add a line with myObject.LocX = 30 and press ALT+P while the mouse cursor is in the Text Editor. The object will jump to the position 30 in the X axis.
To align objects with scripts, we have to first get the properties of several object from a selection, and not just one object. There is a useful command called GetSelected(), which generated a list of objects from a selection. We can create another object to hold the selection list. It will look like this:
- import Blender
- myObject = Blender.Object.Get(‘Cube')
- selectionList = Blender.Object.GetSelected()
If we select any object and run the script, the list will be created with the name of all objects. The objects in the list can be displayed on the auxiliary window with for loop. In Python we create for loops by pointing and variable that will be used to repeat a declaration, based on a number. If we compare the variable with the list, it will repeat the declaration for each object in the list. Here is how it works, add a line with for i in selectionList:. And in the next line, add a print i. The i variable will be replaced with the name of the object in each loop interaction.
- import Blender
- myObject = Blender.Object.Get(‘Cube')
- selectionList = Blender.Object.GetSelected()
- for i in selectionList:
- print i
Select a couple of objects and run the script. The auxiliary window will display the name of all selected objects.
We now have all selected objects placed in a list! But, how can we align them all? We will now set the LocY position of all selected objects to be exactly the same as the Cube, assigned as the myObject variable. Replace the print I with an i.LocY = myObject.LocY and this will change the LocY coordinate of all selected objects to be exactly the same as the object pointed in the myObject variable. To update the 3D View, we have to add a Redraw at the end of the script.
- import Blender
- myObject = Blender.Object.Get(‘Cube')
- selectionList = Blender.Object.GetSelected()
- for i in selectionList:
- i.LocY = myObject.LocY
- Blender.Redraw()
Select a couple of objects and run the script. You will see all selected objects to be aligned in the Y axis with the object pointed in the myObject variable.
Change the name of the object assigned to the myObject variable to align the selection with other models. This is a very simple script, but it could be used as a starting point to learn Python and improve your workflow in architectural modeling. In future articles, I will show how to distribute the objects with the same measurements between them and create a simple interface to set options.
Just remember, you have to get Python installed to use the examples showed in this tutorial. I'm using Python 2.6.2 with Blender 3d 2.49b.
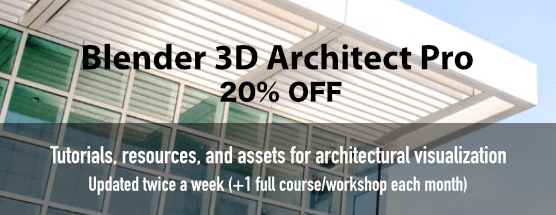
Comments
Jezzabetz
Thankyou so much, ive been wanting to do more python but i just couldn’t figure it out!
Michael A. Beaver
It’s time to update this short article to reflect the changes using Blender 2.5 and Python 3.1.